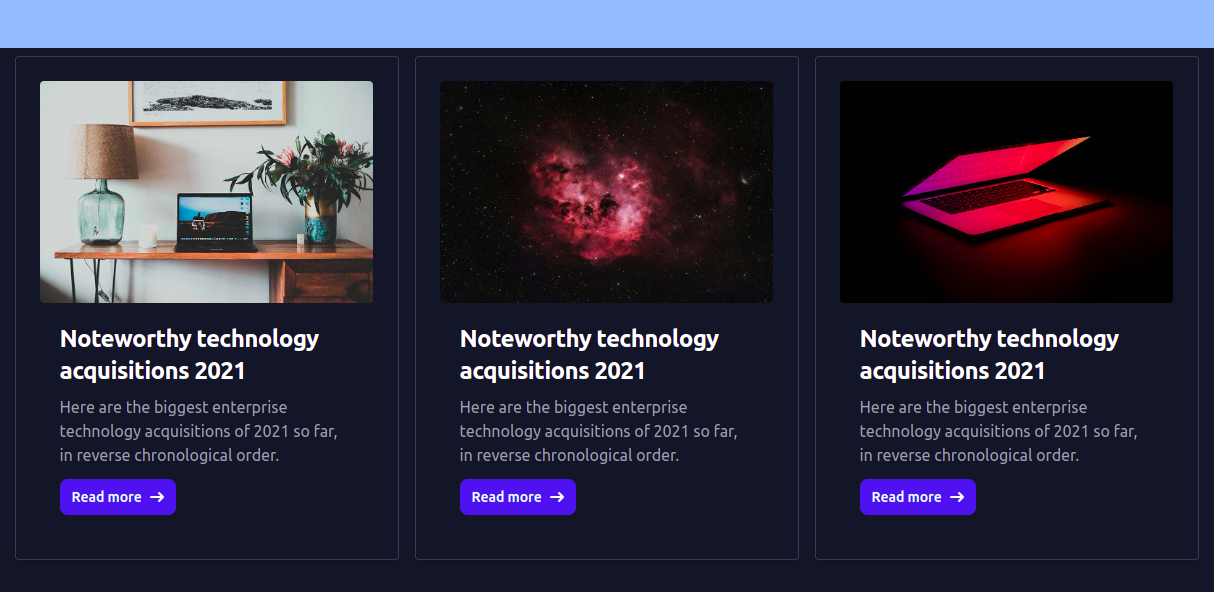
Micro frontends is an architectural style where a large frontend application is split into smaller, independent sections. Each section is developed, deployed, and maintained separately, allowing different teams to work on different parts of the frontend. This approach improves scalability, flexibility, and makes it easier to update or replace individual parts without affecting the whole system.
Let me describe what I did.
In this React app, the Projects
component is exposed using Vite Plugin Federation:
return {
plugins: [
react(),
federation({
name: "remote_projects",
filename: "remoteEntry.js",
exposes: {
"./Projects": "./src/components/Projects",
},
shared: ["react", "react-dom"],
}),
],
preview: {
port: env.VITE_PREVIEW_PORT,
},
server: {
port: env.VITE_DEV_PORT,
},
build: {
modulePreload: false,
target: "esnext",
minify: false,
cssCodeSplit: false,
},
};
This configuration is for the remote
React application that exposes the Projects
component.
For the main
React application, we configure it to load the Projects
component from the remote app:
return {
plugins: [
react(),
federation({
name: "portfolio",
remotes: {
remoteProjects: `${env.VITE_PROJECTS_HOST}/assets/remoteEntry.js`,
},
shared: ["react", "react-dom"],
}),
],
preview: {
port: env.VITE_PREVIEW_PORT,
},
server: {
port: env.VITE_DEV_PORT,
},
build: {
modulePreload: false,
target: "esnext",
minify: false,
cssCodeSplit: false,
},
};
In this setup, the main app
renders the Projects
component from remoteProjects
, which is defined in the Vite configuration. This means that the Projects
component is loaded from another React application.
...
const Projects = React.lazy(() => import("remoteProjects/Projects"));
...
function App() {
return (
<>
<NavBar />
<Suspense fallback={<Skeleton />}>
<ErrorBoundary
fallback={
<SomethingWentWrong message="Projects Micro Frontend Team might be on a Vacation" />
}
>
<Projects />
</ErrorBoundary>
</Suspense>
<span>Tech</span>
</>
);
}
This code leverages React.lazy
for code-splitting and Suspense
for loading the remote Projects
component, with an ErrorBoundary
to handle errors gracefully.
🚀 Check out this simple app implementing the micro frontend concept: Visit Project 🌐